Effective Guide on Vue components
You cannot create a meaningful Vue app without components. Here's the easy and effective guide to working with components in vuejs
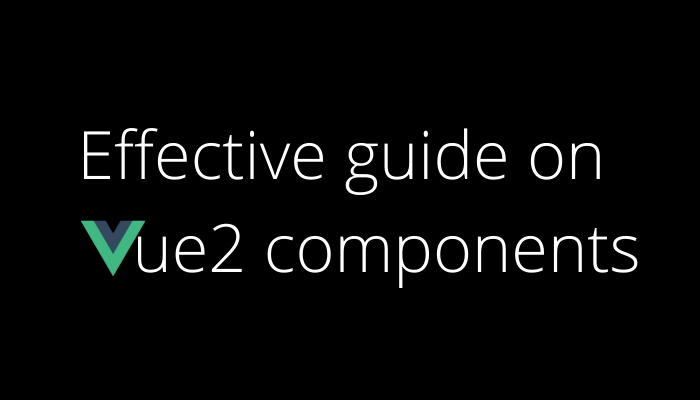
If Vue app is a room, then components are bricks. So you see its very important to learn about Vue components. Here I will discuss basic concepts around Vue.js components and examples around that.
Setup for this guide
Component is a building block of any Vue app. Component means small section of the app, which can be re-used anywhere in the app. Any normal Vue component consists of HTML UI (template), script and CSS. We'll give you example shortly.
In order to follow along, create a new app using vue create
command as follows
vue create vue-component-example
Open the project using your favorite IDE. Mine is VS Code :)
Once you open your project you might see the following directory structure.
App.vue
is your root component which is imported in main.js
. Now we have to write our components on top of it.
You can also notice HelloWorld.vue
but this is sample file. You can safely delete it for this guide.
Also remove reference of HelloWorld
component from App.vue
file.
Here are the lines you want to remove
<HelloWorld msg="Welcome to Your Vue.js App"/> //remove this
.
.
.
import HelloWorld from './components/HelloWorld.vue' // remove this
Writing your first Vue component
Let's take an example where we want to display Project information in a card using component.
We will create a file called ProjectInfo.vue
under components directory.
Inside that let's write our vue component skeleton with 3 parts i.e. html template, script and style.
If you have octref.vetur
extension installed, its intellisense will do the magic for you like the screenshot below.
Here's what you need
<template>
//html goes here
</template>
<script>
export default {
//javascript goes here
}
</script>
<style scoped>
//css specific to your component
</style>
Write some HTML inside the template e.g.
<div>
Project Component.
</div>
This is simplest thing you can do with the component.
Now import it in App.vue
file using import statement.
import ProjectInfo from './components/ProjectInfo.vue';
Next you need to register this component in components section of App.vue
. You can do this by adding it to components section.
components: {
ProjectInfo
}
So if you want to use component A inside component B. Then you need to register component A inside B, along with all other components you want to use in B.
components
is a property of any vue component with type Object. Here's how it would look with multiple components.
components:{
ComponentA,
ComponentB,
"component-c":ComponentC
}
Notice how we can re-name the reference of registered component using JS Object Notation.
Once you have registered, now you can use the component, simply like any other HTML element. e.g.
<ProjectInfo />
you can also write it as
<project-info />
Run the project if not running (npm run serve
)
You'll see something like this in browser
You can call the component tag as many times as you like. This helps us to re-use the blocks of the page (just like the concept of Web Component)
Parts of components
There 3 main parts of components
Template
This is basically the HTML markup of the component. Any component can use other components as well (after importing and registering).
Script
This is the Javascript section of the component. It contains the definition of the component. It can also contain the functions and normal JS code.
CSS / Style
You can write style definition specific to your vue component. Don't miss the scoped
property on style tag.
How to compose using Components
Now you know, how to create components. But how do you compose your components? If you are given a functionality how will you convert that into bunch of components? How will you define scope of each component?
There is no definite answer. Here are few points which might help.
- Each div in the page can be viewed as separate vue component.
- If you can name a section on the page, you can get component out of it.
- You also need to consider if there is some section / design is repeating on the UI, there's a good chance then you can create 1 component and re-use.
These are not rules, just few pointers which might help. You'll get better at it with experience, I promise :)
Register components
In order to use a component, you have to register it. There are 2 common ways.
Local Registration
We already used it in the example above. We need to manually import each component we want to use inside current component, then add it to components
object of the definition.
This way is preferred when we want to encapsulate the use of particular functionality and we don't want to pollute the global namespace of the project.
For Example, if you are building an eCommerce Store, it makes sense to locally register PaymentDetails
component in CheckoutPage
component.
Global Registration
This method is normally used with components which are needed on most of the app. e.g. UI Components.
Suppose if you have built a custom Switch Button in your app and encapsulated it in my-switch
component. Then It would be tedious to import it on all pages which need this component. So better register it globally.
You can do this by using Vue.component('my-component',MyComponent)
statement.
Let's create an example global component in our project called GlobalComponent
. We will just keep an <h1>
tag inside it and we would use it without explicitly registering it.
Create the component GlobalComponent.vue
inside components directory.
Add the <h1>
tag with some text to template of this component.
In order to register it globally, import it to main.js
file and use Vue.component
. See snippet below.
import GlobalComponent from './components/GlobalComponent'
Vue.component('global-component',GlobalComponent);
That's it. Now you can use it anywhere in the Vue app. Try it out in App.vue
or even ProjectInfo.vue
Note- don't overuse global registration else your app might load slow first time.
Props
Props make components all the more powerful. Props help you to pass data to the component. (You can call them properties.)
They help component to act dynamically. Let's continue with ProjectInfo
component example.
Let's say each Project
has these properties title
, allocatedEmployeeCount
, startDate
for simplicity.
Now we want to modify the component such that it takes these properties as input props
and display them.
First let's fix the html markup.
<template>
<div class="row">
<h1>Project Alpha</h1>
<p><strong>Employees Allocated:</strong> 100</p>
<p><strong> Start Date : </strong> 29-02-2021</p>
</div>
</template>
<script>
export default {
name:'ProjectInfo',
}
</script>
<style scoped>
</style>
We'll keep things simple for this guide.
Now we'll add props to this component.
Add props
property to component definition alongside Component name
.
export default {
name:'ProjectInfo',
props:['title','allocatedEmployeeCount','startDate']
}
Now replace the placeholder text in markup with these fields, just like you would do for any data property. e.g.
<div class="row">
<h1>{{title}}</h1>
<p><strong>Employees Allocated:</strong> {{allocatedEmployeeCount}}</p>
<p><strong> Start Date : </strong> {{startDate}}</p>
</div>
If see output, it would have blank spaces now, because we haven't really passed the actual values to props inside App.vue
Now inside App.vue
, pass the actual values like this.
<project-info title="Project Alpha" allocatedEmployeeCount="100" startDate="29-01-2021"/>
Now you can see the output.
You can also pass props dynamically using data properties of the component like this. Notice the :
in front of prop name.
<project-info :title="title" :allocatedEmployeeCount="allocatedEmployeeCount" :startDate="startDate"/>
You can also define the data-type of props explicitly like this.
props: { title: String, allocatedEmployeeCount: Number, startDate: String },
This will ensure that you can only pass valid props. More on this in some other post.
Component properties
Vue components have many properties. Some useful ones are mentioned below.
- data
- computed
- methods
- mixins
- name
- components
More on these in some other post.
Conclusion
In this post, you learnt about basic building block of any Vue app, Components. Now you can go ahead and try all of this. Ask any queries on Twitter @MohitSehgl.
You can also check the whole code on this Github repo.
Happy Coding.
WRITTEN BY
Mohit Sehgal
Want to positively impact the world. Underrated Altruist, Full Stack Developer, MEVN Stack (MongoDB, Express.js, Vue.js, Node.js)