Important Interview questions for ExpressJS / Node.JS
Node.JS is among the world’s top 5 most loved frameworks. ExpressJS is undoubtedly the most used Node.JS framework. So you might be interested in some vacancies around the globe with a profile in NodeJS/ExpressJS. In this post, I will be posting a few very important Interview questions. I hope it helps.
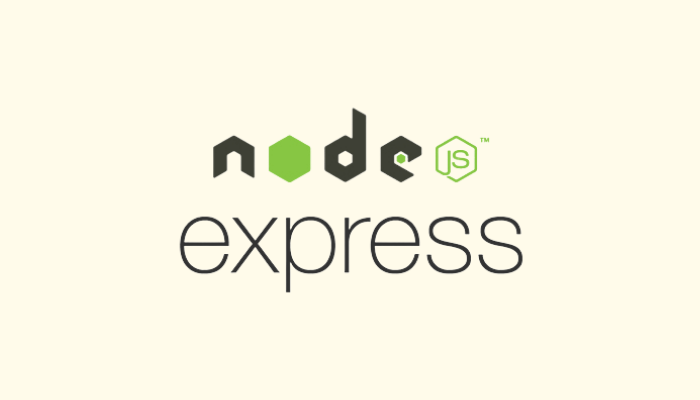
Node.JS is among the world’s top 5 most loved frameworks. ExpressJS is undoubtedly the most used Node.JS framework. So you might be interested in some vacancies around the globe with a profile in NodeJS/ExpressJS. In this post, I will be posting a few very important Interview questions. I hope it helps.
What is NodeJS?
Node.JS is a server-side Javascript runtime platform built on the top of Google’s V8 Engine. It is asynchronous and event-driven and is designed to build scalable network applications. Node.JS is written in C++, it's lightweight and extensively used in various kinds of enterprise software.
What is ExpressJS?
Express.JS is the web application framework built on top of Node.JS. You can build a complete web app’s backend i.e REST APIs, DB Interactions, serve files, create realtime apps, cron jobs, etc. You can install it using a single command.
npm install express --save
What are the core features of ExpressJS?
Express.JS is packed with various features like :
- Rest APIs support(you can build Rest API in minutes),
- Support for NoSQL out of the box,
- Routing,
- Middleware,
- Debugging,
- Templating (like Jade, EJS, Handlebars),
- Serve Static Assets (like HTML, CSS, Images, and videos)
- Support for realtime apps using SocketIO.
There are many more but these are the base of any strong web-app.
How can we create a Rest API route in ExpressJS?
There are 2 ways of going about it:
Using App Object
You get the Express App Object like this
const app = express();
Then you can call app.{{http_method}}, where http_method is any among GET
, POST
, PUT
, DELETE
, and other standard HTTP methods. A simple GET route can be like this.
app.get('/some/path', function (req,res){
res.send('Hello Express!!');
});
Using Router Object
- Get the router object
const express = require('express')
const router = express.Router()
- Create the route using a
router.{{http_method}}
.
router.post(
'/login',
// This function is called Router Function
function (req,res){
//write the logic
res.send('Login Succes');
});
- Mount the route on the express app.
const app = express();
app.use('/',router);
Using a router is better to practice as compared to directly using the app.
What is a router function?
The router function is a function that handles the request on a given express route.
What is middleware in ExpressJS?
Middleware is a function that runs on the HTTP request before the request reaches the router function.
You can register a middleware using the app.use
method.
Some common use-cases of middleware are converting the request object into JSON format, authenticating the request using tokens in headers or cookies, limiting the number of requests from a given client.
There can be multiple middlewares in one Express App. Each middleware can be bind to a few or all the routes based on the registration of middleware.
What are the parameters of middleware in ExpressJS?
If you want to create custom middleware then it has 3 parameters i.e. req, res, and next.
//express middleware
app.use(function(req,res,next){
// middleware logic goes here
// call next once the job of middleware is done
next();
});
req is the request object which represents the Http request.
res is the response object which represents the response, if middleware returns response then the router function will not be called.
next is the function which represents the next function in the sequence. It is called when current middleware is done with its thing and is ready to pass on the request to the next middleware or router function.
What is mongoose?
Mongoose is ODM (Object Data Modelling) library that helps our Express App to connect to MongoDB using Schemas. It provides us the elegant way of writing business logic or data access logic.
Here You can create Schema classes where you can define data- structure, data-types, validation, and much more.
You can install it using npm.
npm i mongoose --save
What is Token-based Authentication? What is JWT?
It is an authentication mechanism where Token is passed along with the HTTP Requests, either in the body or in headers. These tokens are authenticated before each request is processed. Tokens are generated using JWT (JSON Web Token)
In ExpressJS we use jsonwebtoken
, a secure and well-tested npm module to handle token-based authentication.
You can save the identity info in encrypted format in token using jwt.sign
and then authenticate a token using jwt.verify
. You can also set an expiry time for these tokens.
What is "passport.js"?
Passport.JS is simple and yet feature-heavy authentication middleware for Express.JS. You can build various authentication strategies in your Express app like Facebook, Google, Twitter, Github, LinkedIn, or simple username/password
Strategies mean a way to verify the authentication, like username/password or OAuth providers like Facebook, Google, Github, etc.
How to read input in ExpressJS Routes?
input can be read through req.body or req.query (URL Query). Suppose we have built a login API that accepts username and password. We will make it a post route and we can read the input using req.body. Check Example.
app.post('/login',function(req,res){
const username = req.body.username;
const password = req.body.password;
//do authentication
//send response
res.json({message:'Login successful'});
});
How to validate input in ExpressJS Routes?
You can use very common validation libraries like express-validator
or @hapi/joi
Express-Validator provides a set of utility functions for easy validations
Hapi/Joi is the schema-based validation library, which gives us the ability to validate whether the given input is in the schema’s format or not. It is regarded as the most powerful data validation library for JS.
What is Socket.IO?
Socket.IO is the Node.JS library that allows your app to provide realtime features like Chat, Scores Update, Live Analytics, and much more.
It is a very reliable real-time engine which works on the top of Socket based communication.
You can build a simple chat app using Socket.IO in minutes.
What is Pub-Sub architecture?
It is a software design pattern where the sender publishes the events and subscribers tune-in to the events and acts accordingly.
It has 2 components:
i) Publishers – These are the software components that publish the event. Let’s say, a new user signs up. The publisher module (Authentication) will publish the event with some name like user_signed_up.
ii) Subscribers: Subscribers are the software components that tune in to some events. They want to perform some action if that event happens. Let’s say there is an email sequence that you need to send to every new user. You can create a module called EmailSender, and that can subscribe to an event called user_signed_up. It will be notified whenever a new user signs up (via Authentication publisher), then it will execute its logic.
What is the event loop in Node.JS? What is asynchronous I/O?
The event loop is something that allows Node.JS to perform non-blocking IO. This is the feature that sets Node.JS apart from all other backend platforms.
The event loop utilizes OS Kernel whenever possible to execute the IO at the background and when that process is finished, OS Kernel adds a callback to the poll queue.
If you are interested in learning more about Event Loop in Node.JS, then check this great article by D. Khan. Alternatively, do check out the official guide on the event loop in Node.JS.
What are promises, callbacks? What is the use of async-await?
Callback
Originally there was the concept of callback functions in Node.JS. Callbacks are nothing but normal javascript functions that are executed once an operation is finished.
Suppose you want to read a file in Node.JS, and you want to print content on the screen once you read it.
In Node.JS, you need to call the readFile function of the fs module which accepts a function as the second parameter. So to execute the functionality you need to create a function and pass it with a readFile call. Check the code
var fs = require("fs");
fs.readFile('input.txt', function (err, data) {
if (err) return console.error(err);
console.log(data.toString());
});
This is a classic example of the callback.
Promises
A Promise is an object, which represents an operation whose result will be received in the future.
If that operation is successful then the promise returns the result, if it fails then the promise returns the reason why it failed.
The use of callbacks can make code look very complicated, (which is famously called callback hell). To avoid that we use Promises. All new libraries in Javascript use promises.
Aync
-Await
In the latest versions of Javascript. We can use a combination of aysnc
/await
. It is just a better syntax to work with promises.
The async
keyword can be placed before any javascript function which means, this function will always return a promise. Even if you return any non-promise value like Object, Integer, string, that would be wrapped around a promise automatically.
await
is the keyword that works only in functions that are marked async
. The use of the keyword await makes javascript to wait until the promise is resolved or rejected totally (has result or error).
For more read this simple and great post on async-await.
Conclusion
These are just a few very important questions which you must know. Otherwise, there are tons of other things that can be asked in an Express.JS /Node.JS interview. I will try and write more posts soon.
If you are hiring Node.JS developers, you may love this guide
Do you have any specific questions to ask? Feel free to ask in Twitter DM @MohitSehgl.
WRITTEN BY
Mohit Sehgal
Want to positively impact the world. Full Stack Developer, MEVN Stack (MongoDB, Express.js, Vue.js, Node.js)